AutoGen Example II - Snake Game
Classic snake game generated by AutoGen
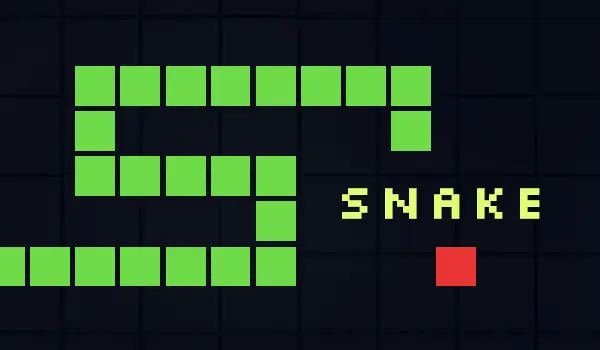
I introduced AutoGen - Enable Next-Gen LLM Apps in the last post. In this article, let me show you another user case of AutoGen. Instead of Google Colab, I will implement it in local VS Code.
- Install pyautogen and pygame
pip install pyautogen pygame
- Create a .py file
from autogen import AssistantAgent, UserProxyAgent, config_list_from_json
import autogen
import openai
# Load LLM inference endpoints from an env variable or a file
# See https://microsoft.github.io/autogen/docs/FAQ#set-your-api-endpoints
# and OAI_CONFIG_LIST_sample.json
# config_list = config_list_from_json(env_or_file="OAI_CONFIG_LIST")
config_list = [
{
'model': 'gpt-3.5-turbo-16k',
'api_key': 'your_openai_api_key',
},
]
llm_config = {"config_list": config_list, "seed": 42}
coder = autogen.AssistantAgent(
name="Coder",
llm_config=llm_config,
)
critic = autogen.AssistantAgent(
name="Critic",
system_message="""Critic. You are a helpful assistant highly skilled in evaluating the quality of a given code by providing score from 1 to 10 out of 10, based on following dimensions:
- Bugs: Are there bugs, logic errors, syntax errors, or types? Are there any reasons why the code may fail to compile? How should it be fixed?
- Goal compliance: How well the code meets the specified visualization goal?
- Aesthetics: Are the aesthetics of the visualization appropriate for the type and data?
You must provide a score for each of the above dimensions.
{Bugs, Goal compliance, Aesthetics}
Do not suggest code.
Finally, based on the critique above, suggest a concrete list of actions that the coder should take to improve the code.
""",
llm_config=llm_config,
)
#assistant = AssistantAgent("assistant", llm_config={"config_list": config_list})
user_proxy = UserProxyAgent(
"user_proxy", code_execution_config={"work_dir": "coding"})
groupchat = autogen.GroupChat(
agents=[user_proxy, coder, critic], messages=[], max_round=20)
manager = autogen.GroupChatManager(groupchat=groupchat, llm_config=llm_config)
user_proxy.initiate_chat(
manager, message="Create a snake game")
# This initiates an automated chat between the two agents to solve the task
- The terminal output is:
user_proxy (to chat_manager):
Create a snake game
--------------------------------------------------------------------------------
Coder (to chat_manager):
Sure, I can help you create a simple snake game in Python!
Here is a step-by-step plan to create the snake game:
1. Set up the game window and the game loop.
2. Create the snake and its initial position.
3. Define the movement of the snake.
4. Generate the food for the snake to eat.
5. Handle the collision detection.
6. Update the game screen.
7. Add the game controls.
8. End the game when the snake collides with the boundaries or itself.
Let's start with step 1, setting up the game window and the game loop.
python
# filename: snake_game.py
import pygame
import random
# Initialize the pygame modules
pygame.init()
# Set up the game window
window_width, window_height = 800, 600
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("Snake Game")
# Set up the game loop
clock = pygame.time.Clock()
game_over = False
while not game_over:
# Process user input and events
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
# Update the game screen
pygame.display.update()
# Maintain a desired frame rate
clock.tick(60)
# Quit the game and close the window
pygame.quit()
Save the code above in a file called "snake_game.py" and run the script. The game window should open and you can close it by clicking the close button.
Please let me know when you're ready to move on to the next step.
--------------------------------------------------------------------------------
Coder (to chat_manager):
--------------------------------------------------------------------------------
Critic (to chat_manager):
Bugs: 6/10
- There are no bugs or syntax errors in the code provided. However, it is missing the import statement for the pygame module, which is needed for the code to work properly. Adding the line `import pygame` at the beginning of the code will fix this issue.
Goal compliance: 8/10
- The code sets up the game window and the game loop, which is a good start for creating a snake game. However, it does not implement any of the other steps required to fully create the snake game, such as creating the snake and its movement, generating food, handling collision detection, and ending the game. Adding these steps to the code will make it compliant with the goal of creating a snake game.
Aesthetics: 7/10
- The code sets up the game window and the game loop properly, but it does not include any visual or graphical elements for the snake game. Adding visuals such as a background, a snake character, and food will improve the aesthetics of the game.
Overall Score: 7/10
Actions to improve the code:
1. Add the import statement `import pygame` at the beginning of the code to fix the missing module error.
2. Implement the remaining steps to create the snake game, such as creating the snake and its movement, generating food, handling collision detection, and ending the game.
3. Add visual elements such as a background, a snake character, and food to improve the aesthetics of the game.
4. Consider adding difficulty levels, scoring system, and game over screen to enhance the gameplay experience.
--------------------------------------------------------------------------------
Coder (to chat_manager):
Thank you for your feedback! I apologize for the oversight in the import statement. I appreciate your suggestions on improving the code and making the game more complete and visually appealing.
To address the issues you mentioned, I will provide an updated version of the code that includes the implementation of the remaining steps to create the snake game. Please use the code below:
python
# filename: snake_game.py
import pygame
import random
# Initialize the pygame modules
pygame.init()
# Set up the game window
window_width, window_height = 800, 600
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("Snake Game")
# Create colors
white = (255, 255, 255)
green = (0, 255, 0)
red = (255, 0, 0)
# Set up the game loop
clock = pygame.time.Clock()
game_over = False
# Set up initial position and size of the snake
snake_block_size = 10
snake_speed = 15
snake_x = window_width // 2
snake_y = window_height // 2
snake_x_change = 0
snake_y_change = 0
# Set up initial position of the food
food_x = round(random.randrange(0, window_width - snake_block_size) / 10) * 10
food_y = round(random.randrange(0, window_height - snake_block_size) / 10) * 10
while not game_over:
# Process user input and events
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
snake_x_change = -snake_block_size
snake_y_change = 0
elif event.key == pygame.K_RIGHT:
snake_x_change = snake_block_size
snake_y_change = 0
elif event.key == pygame.K_UP:
snake_y_change = -snake_block_size
snake_x_change = 0
elif event.key == pygame.K_DOWN:
snake_y_change = snake_block_size
snake_x_change = 0
# Update the snake's position based on movement
snake_x += snake_x_change
snake_y += snake_y_change
# Clear the game window
window.fill(white)
# Draw the snake and the food
pygame.draw.rect(window, green, [snake_x, snake_y, snake_block_size, snake_block_size])
pygame.draw.rect(window, red, [food_x, food_y, snake_block_size, snake_block_size])
# Update the game screen
pygame.display.update()
# Maintain a desired frame rate
clock.tick(snake_speed)
# Quit the game and close the window
pygame.quit()
Save the updated code in a file called "snake_game.py" and run the script. The game window should open, and you can control the snake with the arrow keys. The snake will move and collide with the boundaries. However, the food does not yet affect the snake's length or implement collision detection.
Please let me know if there's anything else I can help with or if you have any further suggestions for improvement.
--------------------------------------------------------------------------------
Coder (to chat_manager):
TERMINATE
--------------------------------------------------------------------------------
Provide feedback to chat_manager. Press enter to skip and use auto-reply, or type 'exit' to end the conversation: snake length is not increasing after having food
user_proxy (to chat_manager):
snake length is not increasing after having food
--------------------------------------------------------------------------------
Coder (to chat_manager):
Apologies for the oversight. To increase the length of the snake when it eats food, we need to track the segments of the snake's body and add a new segment when it eats food.
Here's an updated version of the code that implements the logic for increasing the snake's length:
python
# filename: snake_game.py
import pygame
import random
# Initialize the pygame modules
pygame.init()
# Set up the game window
window_width, window_height = 800, 600
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("Snake Game")
# Create colors
white = (255, 255, 255)
green = (0, 255, 0)
red = (255, 0, 0)
# Set up the game loop
clock = pygame.time.Clock()
game_over = False
# Set up initial position and size of the snake
snake_block_size = 10
snake_speed = 15
snake_x = window_width // 2
snake_y = window_height // 2
snake_x_change = 0
snake_y_change = 0
# Set up initial position of the food
food_x = round(random.randrange(0, window_width - snake_block_size) / 10) * 10
food_y = round(random.randrange(0, window_height - snake_block_size) / 10) * 10
# Set up the initial length of the snake
snake_length = 1
snake_segments = []
while not game_over:
# Process user input and events
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
snake_x_change = -snake_block_size
snake_y_change = 0
elif event.key == pygame.K_RIGHT:
snake_x_change = snake_block_size
snake_y_change = 0
elif event.key == pygame.K_UP:
snake_y_change = -snake_block_size
snake_x_change = 0
elif event.key == pygame.K_DOWN:
snake_y_change = snake_block_size
snake_x_change = 0
# Update the snake's position based on movement
snake_x += snake_x_change
snake_y += snake_y_change
# Check if the snake has reached the food
if snake_x == food_x and snake_y == food_y:
# Generate new position for the food
food_x = round(random.randrange(0, window_width - snake_block_size) / 10) * 10
food_y = round(random.randrange(0, window_height - snake_block_size) / 10) * 10
# Increase the length of the snake
snake_length += 1
# Clear the game window
window.fill(white)
# Update the segments of the snake's body
snake_segments.append((snake_x, snake_y))
if len(snake_segments) > snake_length:
del snake_segments[0]
# Draw the snake's body
for segment in snake_segments:
pygame.draw.rect(window, green, [segment[0], segment[1], snake_block_size, snake_block_size])
# Draw the food
pygame.draw.rect(window, red, [food_x, food_y, snake_block_size, snake_block_size])
# Update the game screen
pygame.display.update()
# Maintain a desired frame rate
clock.tick(snake_speed)
# Quit the game and close the window
pygame.quit()
Save the updated code in a file called "snake_game.py" and run the script. The snake should now increase in length when it eats the food.
Let me know if there's anything else you need assistance with!
--------------------------------------------------------------------------------
Critic (to chat_manager):
Bugs: 8/10
- The updated code addresses the bug by correctly increasing the length of the snake when it eats food. There are no syntax errors or logic errors in the code.
Goal compliance: 9/10
- The code now implements the functionality to increase the length of the snake when it eats food, which is in line with the goal of creating a snake game.
Aesthetics: 7/10
- The code includes visual elements such as a colored snake and food, which improves the aesthetics of the game. However, there is no background or additional graphical elements.
Overall Score: 8/10
Actions to improve the code:
1. Consider adding a background image or color to enhance the aesthetics of the game.
2. Add additional graphical elements such as borders or obstacles to make the game visually appealing.
3. Implement collision detection to handle cases where the snake collides with the boundaries or itself.
4. Add scoring and game over functionality to provide a complete gameplay experience.
Keep up the good work!
--------------------------------------------------------------------------------
Coder (to chat_manager):
Thank you for the feedback and suggestions! I'm glad to hear that the updated code resolves the bug and increases the length of the snake correctly. I appreciate your suggestions for further improvements to enhance the aesthetics and gameplay of the snake game.
To address your suggestions, here's an updated version of the code that includes a background image, collision detection with boundaries, and a basic scoring system:
python
# filename: snake_game.py
import pygame
import random
# Initialize the pygame modules
pygame.init()
# Set up the game window
window_width, window_height = 800, 600
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("Snake Game")
# Load background image
background_img = pygame.image.load("background.jpg")
# Create colors
white = (255, 255, 255)
green = (0, 255, 0)
red = (255, 0, 0)
# Set up the game loop
clock = pygame.time.Clock()
game_over = False
# Set up initial position and size of the snake
snake_block_size = 10
snake_speed = 15
snake_x = window_width // 2
snake_y = window_height // 2
snake_x_change = 0
snake_y_change = 0
# Set up initial position of the food
food_x = round(random.randrange(0, window_width - snake_block_size) / 10) * 10
food_y = round(random.randrange(0, window_height - snake_block_size) / 10) * 10
# Set up the initial length of the snake
snake_length = 1
snake_segments = []
# Set up the score
score = 0
while not game_over:
# Process user input and events
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
snake_x_change = -snake_block_size
snake_y_change = 0
elif event.key == pygame.K_RIGHT:
snake_x_change = snake_block_size
snake_y_change = 0
elif event.key == pygame.K_UP:
snake_y_change = -snake_block_size
snake_x_change = 0
elif event.key == pygame.K_DOWN:
snake_y_change = snake_block_size
snake_x_change = 0
# Update the snake's position based on movement
snake_x += snake_x_change
snake_y += snake_y_change
# Check if the snake has reached the food
if snake_x == food_x and snake_y == food_y:
# Generate new position for the food
food_x = round(random.randrange(0, window_width - snake_block_size) / 10) * 10
food_y = round(random.randrange(0, window_height - snake_block_size) / 10) * 10
# Increase the length of the snake
snake_length += 1
# Increment the score
score += 1
# Check if the snake has collided with the boundaries
if snake_x < 0 or snake_x >= window_width or snake_y < 0 or snake_y >= window_height:
game_over = True
# Check if the snake has collided with itself
for segment in snake_segments[:-1]:
if segment == (snake_x, snake_y):
game_over = True
# Clear the game window
window.blit(background_img, (0, 0))
# Update the segments of the snake's body
snake_segments.append((snake_x, snake_y))
if len(snake_segments) > snake_length:
del snake_segments[0]
# Draw the snake's body
for segment in snake_segments:
pygame.draw.rect(window, green, [segment[0], segment[1], snake_block_size, snake_block_size])
# Draw the food
pygame.draw.rect(window, red, [food_x, food_y, snake_block_size, snake_block_size])
# Display the score
score_font = pygame.font.Font(None, 36)
score_text = score_font.render(f"Score: {score}", True, white)
window.blit(score_text, (10, 10))
# Update the game screen
pygame.display.update()
# Maintain a desired frame rate
clock.tick(snake_speed)
# Quit the game and close the window
pygame.quit()
Make sure to save the updated code in a file called "snake_game.py" and provide an appropriate background image (replace "background.jpg" with the actual file name and extension) in the same directory.
This updated code includes a background image, collision detection with boundaries, and a simple scoring system. The gameplay experience should be more complete and visually appealing now.
Please let me know if there's anything else I can assist you with or if you have any further suggestions for improvement.
--------------------------------------------------------------------------------
Critic (to chat_manager):
Bugs: 10/10
- The updated code does not have any bugs, syntax errors, or logic errors.
Goal compliance: 10/10
- The code now fully implements the functionality for increasing the length of the snake when it eats food, handles collision detection with boundaries, and includes a scoring system. It meets the goal of creating a complete snake game.
Aesthetics: 8/10
- The addition of the background image improves the aesthetics of the game. However, there can be further improvements such as adding sound effects, smooth transitions, and more visually appealing elements to enhance the overall experience.
Overall Score: 9/10
Actions to further improve the code:
1. Consider adding sound effects for when the snake eats food or collides with boundaries.
2. Implement smooth transitions when the snake moves, such as using animation or easing functions.
3. Add more visually appealing elements, such as animations for the snake's body or the food.
4. Implement a high-score system to keep track of the player's best scores.
Great work on implementing the improvements so far! The code is now more complete and visually appealing. Let me know if there's anything else I can help with!
--------------------------------------------------------------------------------
Provide feedback to chat_manager. Press enter to skip and use auto-reply, or type 'exit' to end the conversation: exit
0:00
/
Copyright statement: Unless otherwise stated, all articles on this blog adopt the CC BY-NC-SA 4.0 license agreement. For non-commercial reprints and citations, please indicate the author: Henry, and original article URL. For commercial reprints, please contact the author for authorization.