Embarking on a Symphony of Intelligence: Unveiling CrewAI's Pinnacle in Autonomous Agent Orchestration
Step into the future with CrewAI, where a tapestry of autonomous AI agents converges in a harmonious ballet of collaborative intelligence, redefining the boundaries of seamless teamwork in tackling intricate challenges.
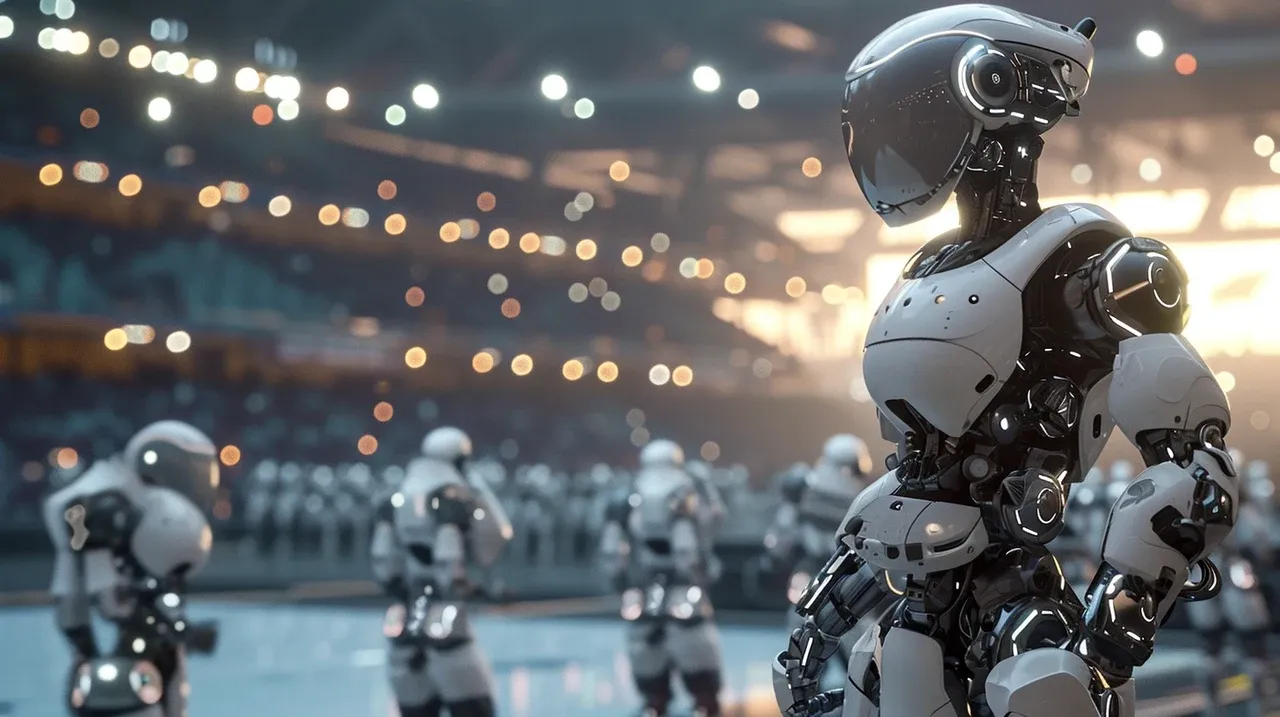
CrewAI is a cutting-edge framework for orchestrating role-playing, autonomous AI agents. By fostering collaborative intelligence, CrewAI empowers agents to work together seamlessly, tackling complex tasks.
Installation
- Create and activate conda env
conda create -n crewai python=3.11
conda activate crewai
- Install essentials
pip install crewai
pip install duckduckgo-search
CrewAI Example
In this article, I’ll use the game builder as an example.This project is an example using the CrewAI framework to automate the process of creating simple games. CrewAI orchestrates autonomous AI agents, enabling them to collaborate and execute complex tasks efficiently.
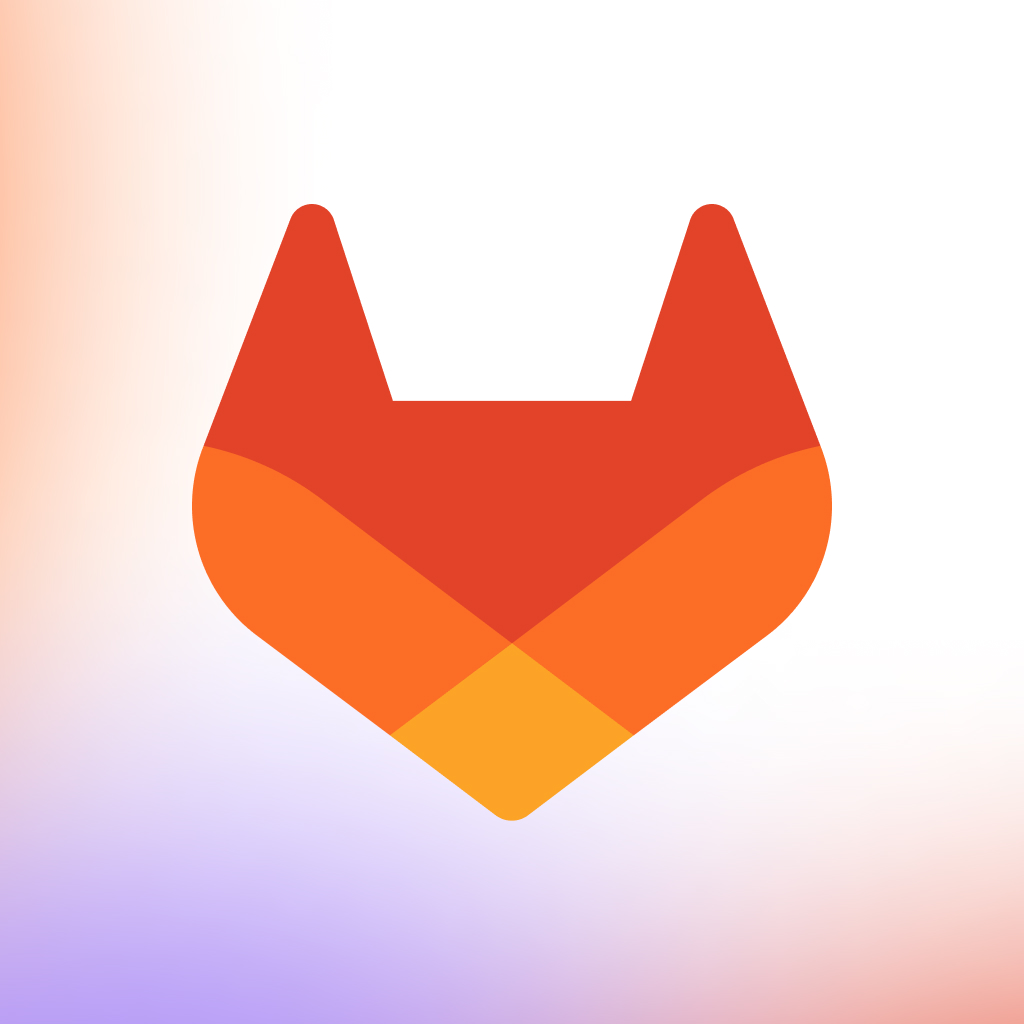
- Clone the repo
git clone http://gitlab.henrywithu.com/henry/crewai-game-builder.git
- Add your OpenAI API key. Rename
.env.example
to.env
OPENAI_API_KEY='your-openai-api-key'
-
Key Components:
./main.py
: Main script file.
./tasks.py
: Main file with the tasks prompts.
./agents.py
: Main file with the agents creation. -
agents.py
from textwrap import dedent
from crewai import Agent
# from langchain.chat_models import ChatOpenAI
from langchain_openai import ChatOpenAI
llm = ChatOpenAI(model='gpt-3.5-turbo') # Loading GPT-3.5
class GameAgents():
def senior_engineer_agent(self):
return Agent(
role='Senior Software Engineer',
goal='Create software as needed',
backstory=dedent("""\
You are a Senior Software Engineer at a leading tech think tank.
Your expertise in programming in python. and do your best to
produce perfect code"""),
allow_delegation=False,
verbose=True
# llm=llm
)
def qa_engineer_agent(self):
return Agent(
role='Software Quality Control Engineer',
goal='create prefect code, by analizing the code that is given for errors',
backstory=dedent("""\
You are a software engineer that specializes in checking code
for errors. You have an eye for detail and a knack for finding
hidden bugs.
You check for missing imports, variable declarations, mismatched
brackets and syntax errors.
You also check for security vulnerabilities, and logic errors"""),
allow_delegation=False,
verbose=True
# llm=llm
)
def chief_qa_engineer_agent(self):
return Agent(
role='Chief Software Quality Control Engineer',
goal='Ensure that the code does the job that it is supposed to do',
backstory=dedent("""\
You feel that programmers always do only half the job, so you are
super dedicate to make high quality code."""),
allow_delegation=True,
verbose=True
# llm=llm
)
- tasks.py
from textwrap import dedent
from crewai import Task
class GameTasks():
def code_task(self, agent, game):
return Task(description=dedent(f"""You will create a game using python, these are the instructions:
Instructions
------------
{game}
Your Final answer must be the full python code, only the python code and nothing else.
"""),
agent=agent
)
def review_task(self, agent, game):
return Task(description=dedent(f"""\
You are helping create a game using python, these are the instructions:
Instructions
------------
{game}
Using the code you got, check for errors. Check for logic errors,
syntax errors, missing imports, variable declarations, mismatched brackets,
and security vulnerabilities.
Your Final answer must be the full python code, only the python code and nothing else.
"""),
agent=agent
)
def evaluate_task(self, agent, game):
return Task(description=dedent(f"""\
You are helping create a game using python, these are the instructions:
Instructions
------------
{game}
You will look over the code to insure that it is complete and
does the job that it is supposed to do.
Your Final answer must be the full python code, only the python code and nothing else.
"""),
agent=agent
)
- main.py
from dotenv import load_dotenv
load_dotenv()
from crewai import Crew
from tasks import GameTasks
from agents import GameAgents
tasks = GameTasks()
agents = GameAgents()
print("## Welcome to the Game Crew")
print('-------------------------------')
game = input("What is the game you would like to build? What will be the mechanics?\n")
# Create Agents
senior_engineer_agent = agents.senior_engineer_agent()
qa_engineer_agent = agents.qa_engineer_agent()
chief_qa_engineer_agent = agents.chief_qa_engineer_agent()
# Create Tasks
code_game = tasks.code_task(senior_engineer_agent, game)
review_game = tasks.review_task(qa_engineer_agent, game)
approve_game = tasks.evaluate_task(chief_qa_engineer_agent, game)
# Create Crew responsible for Copy
crew = Crew(
agents=[
senior_engineer_agent,
qa_engineer_agent,
chief_qa_engineer_agent
],
tasks=[
code_game,
review_game,
approve_game
],
verbose=True
)
game = crew.kickoff()
# Print results
print("\n\n########################")
print("## Here is the result")
print("########################\n")
print("final code for the game:")
print(game)
- Run main.py
Result
Snake is a sub-genre of action video games where the player maneuvers the end of a growing line, often themed as a snake. The player must keep the snake from colliding with both other obstacles and itself, which gets harder as the snake lengthens.
- Input prompt: Snake game, with a score board
- Final code:
import pygame
import time
import random
snake_speed = 15
# Window size
window_x = 720
window_y = 480
# defining colors
black = pygame.Color(0, 0, 0)
white = pygame.Color(255, 255, 255)
red = pygame.Color(255, 0, 0)
green = pygame.Color(0, 255, 0)
blue = pygame.Color(0, 0, 255)
# Initialising pygame
pygame.init()
# Initialise game window
pygame.display.set_caption('GeeksforGeeks Snakes')
game_window = pygame.display.set_mode((window_x, window_y))
# FPS (frames per second) controller
fps = pygame.time.Clock()
# defining snake default position
snake_position = [100, 50]
# defining first 4 blocks of snake body
snake_body = [ [100, 50],
[90, 50],
[80, 50],
[70, 50] ]
# fruit position
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
# setting default snake direction towards
# right
direction = 'RIGHT'
change_to = direction
# initial score
score = 0
# displaying Score function
def show_score(color, font, size):
# creating font object score_font
score_font = pygame.font.SysFont(font, size)
# creating a text surface on which text
# will be drawn
score_surface = score_font.render('Score : ' + str(score), True, color)
# create a rectangular object for the
# text surface object
score_rect = score_surface.get_rect()
# displaying text
game_window.blit(score_surface, score_rect)
# game over function
def game_over(color, font, size):
# creating font object my_font
my_font = pygame.font.SysFont(font, size)
# creating a text surface on which text
# will be drawn
game_over_surface = my_font.render('Your Score is : ' + str(score), True, color)
# create a rectangular object for the
# text surface object
game_over_rect = game_over_surface.get_rect()
# setting position of the text
game_over_rect.midtop = (window_x/2, window_y/4)
# blit will draw the text on screen
game_window.blit(game_over_surface, game_over_rect)
pygame.display.flip()
# after 2 seconds we will quit the
# program
time.sleep(2)
# deactivating pygame library
pygame.quit()
# quit the program
quit()
# Main Function
while True:
# handling key events
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
change_to = 'UP'
if event.key == pygame.K_DOWN:
change_to = 'DOWN'
if event.key == pygame.K_LEFT:
change_to = 'LEFT'
if event.key == pygame.K_RIGHT:
change_to = 'RIGHT'
# If two keys pressed simultaneously
# we don't want snake to move into two
# directions simultaneously
if change_to == 'UP' and direction != 'DOWN':
direction = 'UP'
if change_to == 'DOWN' and direction != 'UP':
direction = 'DOWN'
if change_to == 'LEFT' and direction != 'RIGHT':
direction = 'LEFT'
if change_to == 'RIGHT' and direction != 'LEFT':
direction = 'RIGHT'
# Moving the snake
if direction == 'UP':
snake_position[1] -= 10
if direction == 'DOWN':
snake_position[1] += 10
if direction == 'LEFT':
snake_position[0] -= 10
if direction == 'RIGHT':
snake_position[0] += 10
# Snake body growing mechanism
# if fruits and snakes collide then scores
# will be incremented by 10
snake_body.insert(0, list(snake_position))
if snake_position[0] == fruit_position[0] and snake_position[1] == fruit_position[1]:
score += 10
fruit_spawn = False
else:
snake_body.pop()
if not fruit_spawn:
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
game_window.fill(black)
for pos in snake_body:
pygame.draw.rect(game_window, green,
pygame.Rect(pos[0], pos[1], 10, 10))
pygame.draw.rect(game_window, white, pygame.Rect(
fruit_position[0], fruit_position[1], 10, 10))
# Game Over conditions
if snake_position[0] < 0 or snake_position[0] > window_x-10:
game_over(red, 'times new roman', 90)
if snake_position[1] < 0 or snake_position[1] > window_y-10:
game_over(red, 'times new roman', 90)
# Touching the snake body
for block in snake_body[1:]:
if snake_position[0] == block[0] and snake_position[1] == block[1]:
game_over(red, 'times new roman', 90)
show_score(white, 'times new roman', 20)
# Refresh game screen
pygame.display.update()
# Frame Per Second /Refresh Rate
fps.tick(snake_speed)
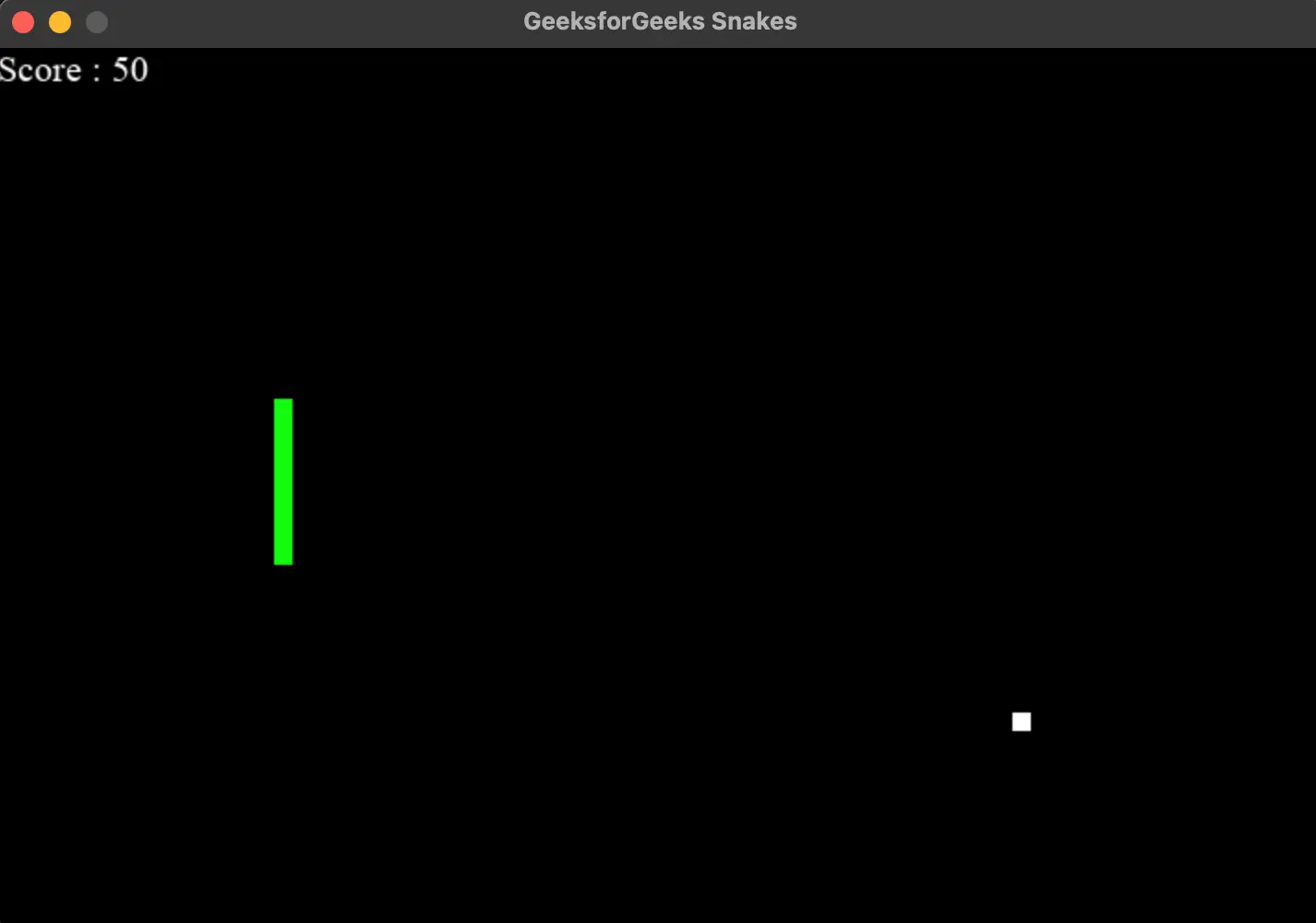
Appendix
If you are interested in AutoGen, here are some related posts:
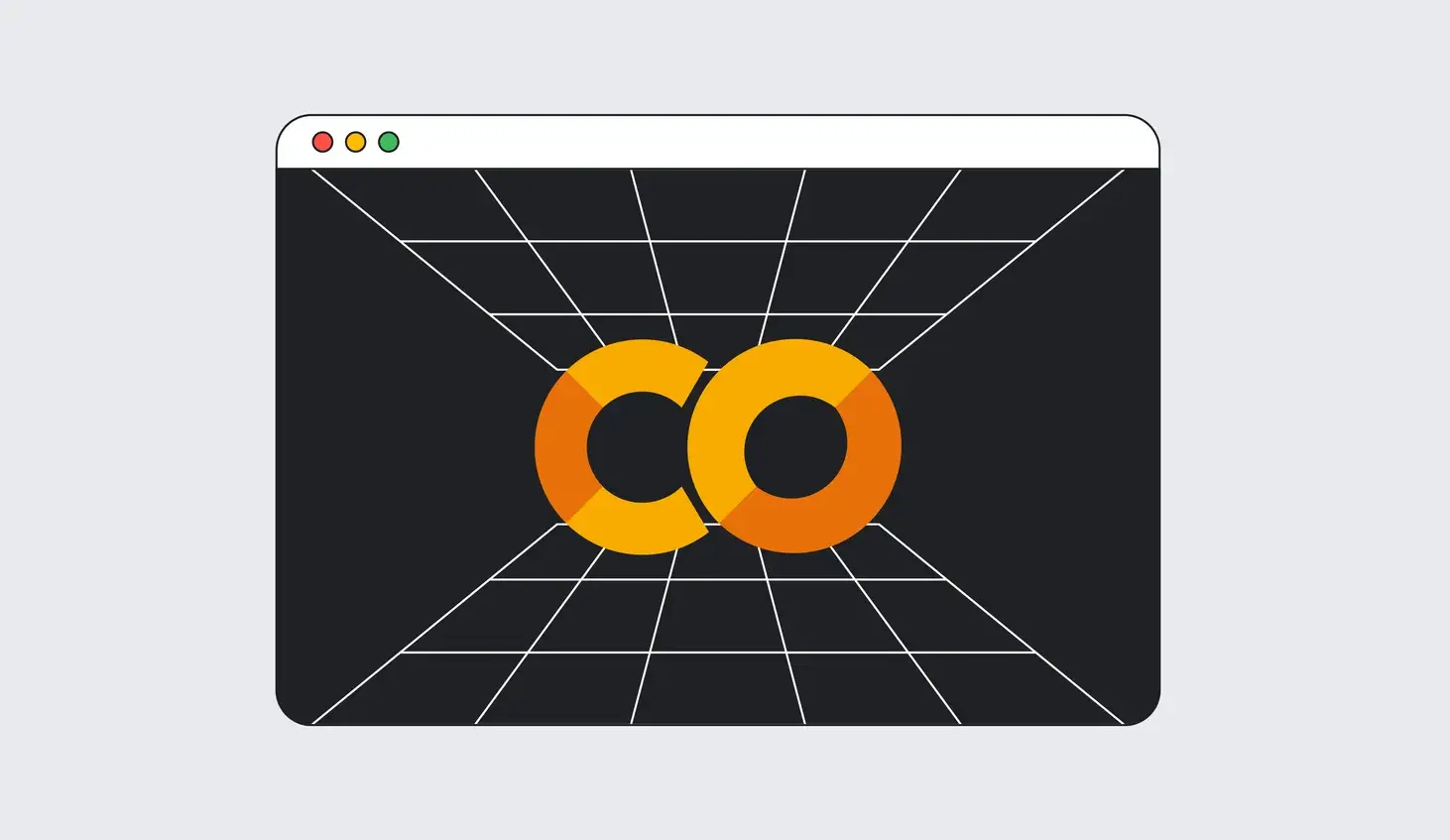
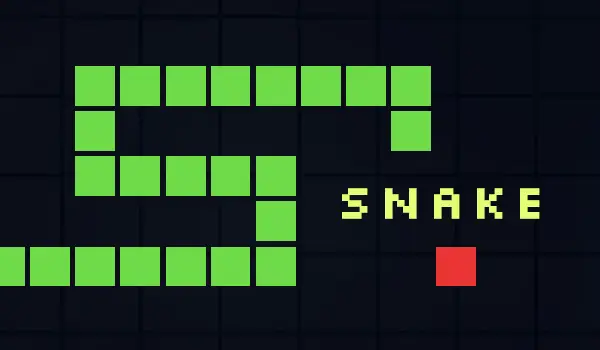
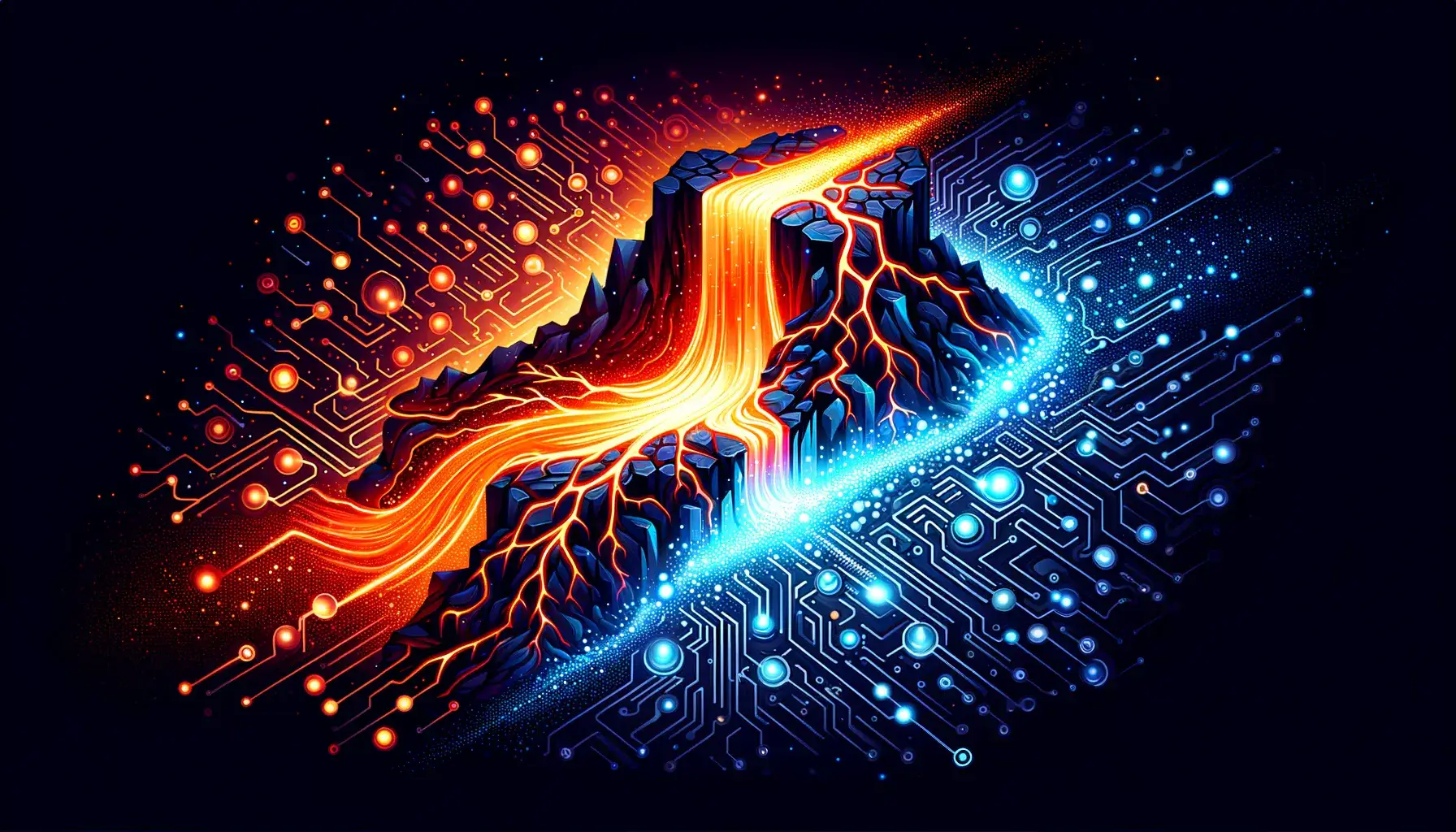
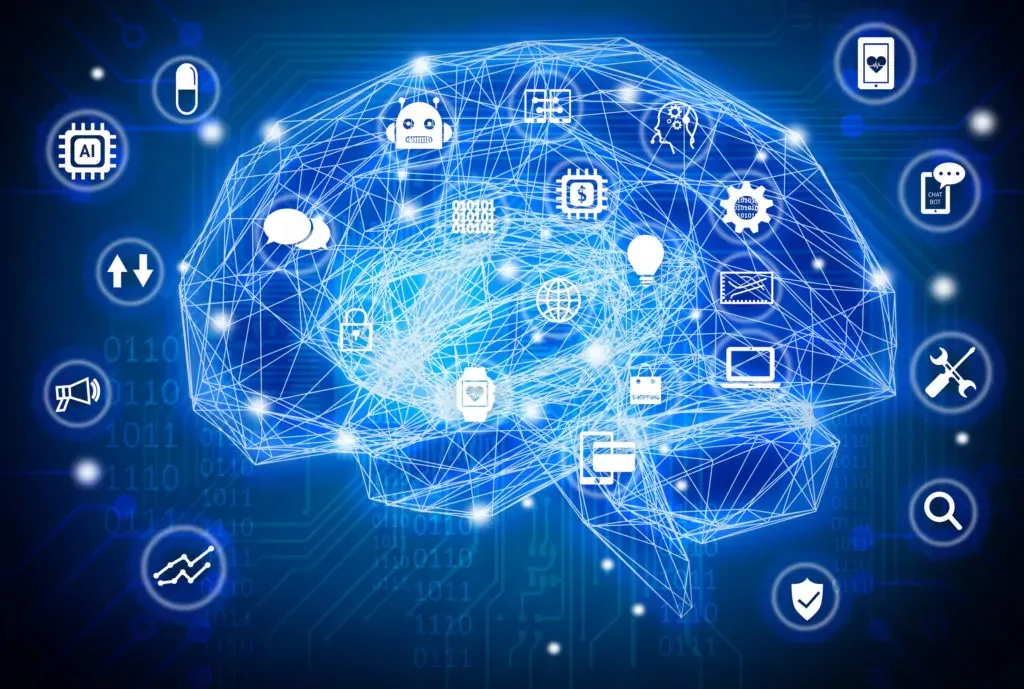
Copyright statement: Unless otherwise stated, all articles on this blog adopt the CC BY-NC-SA 4.0 license agreement. For non-commercial reprints and citations, please indicate the author: Henry, and original article URL. For commercial reprints, please contact the author for authorization.